ZHONGLING TU:
Social Match

(Paper)
2019.6 841mm*594mm Ink-jet printing
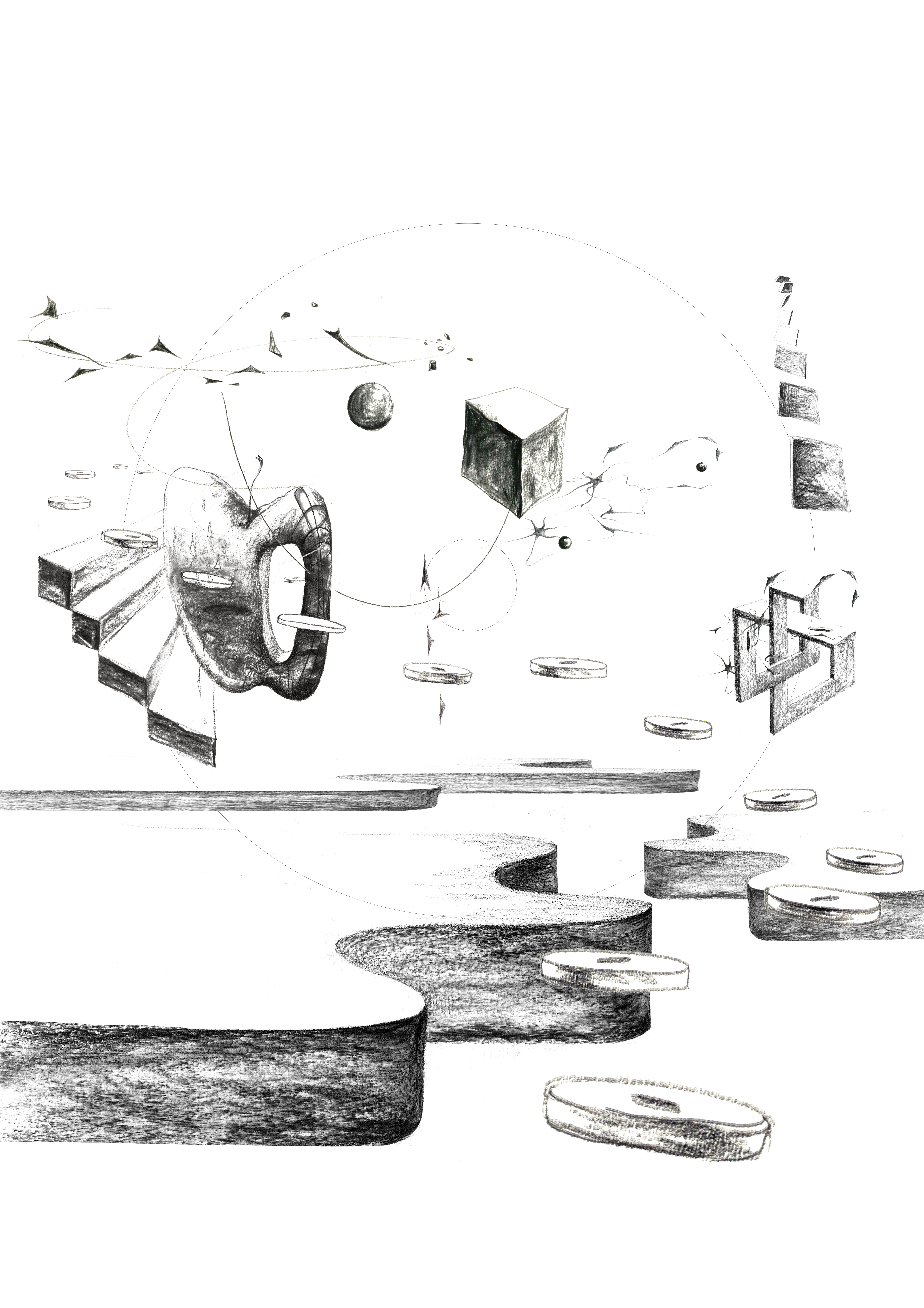
(Paper)
2019.6 841mm*594mm Ink-jet printing
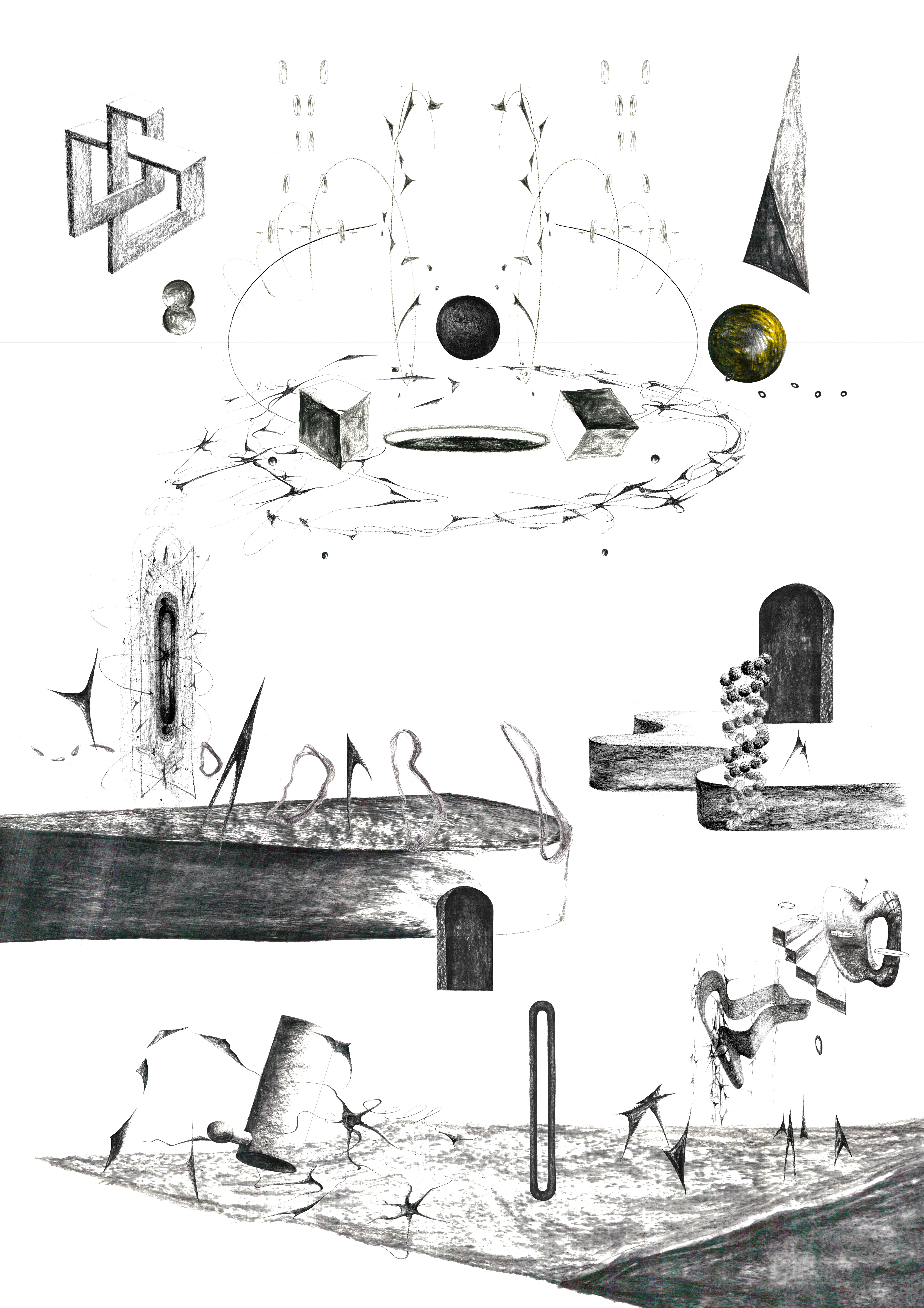
(Paper)
2019.7 841mm*594mm Ink-jet printing
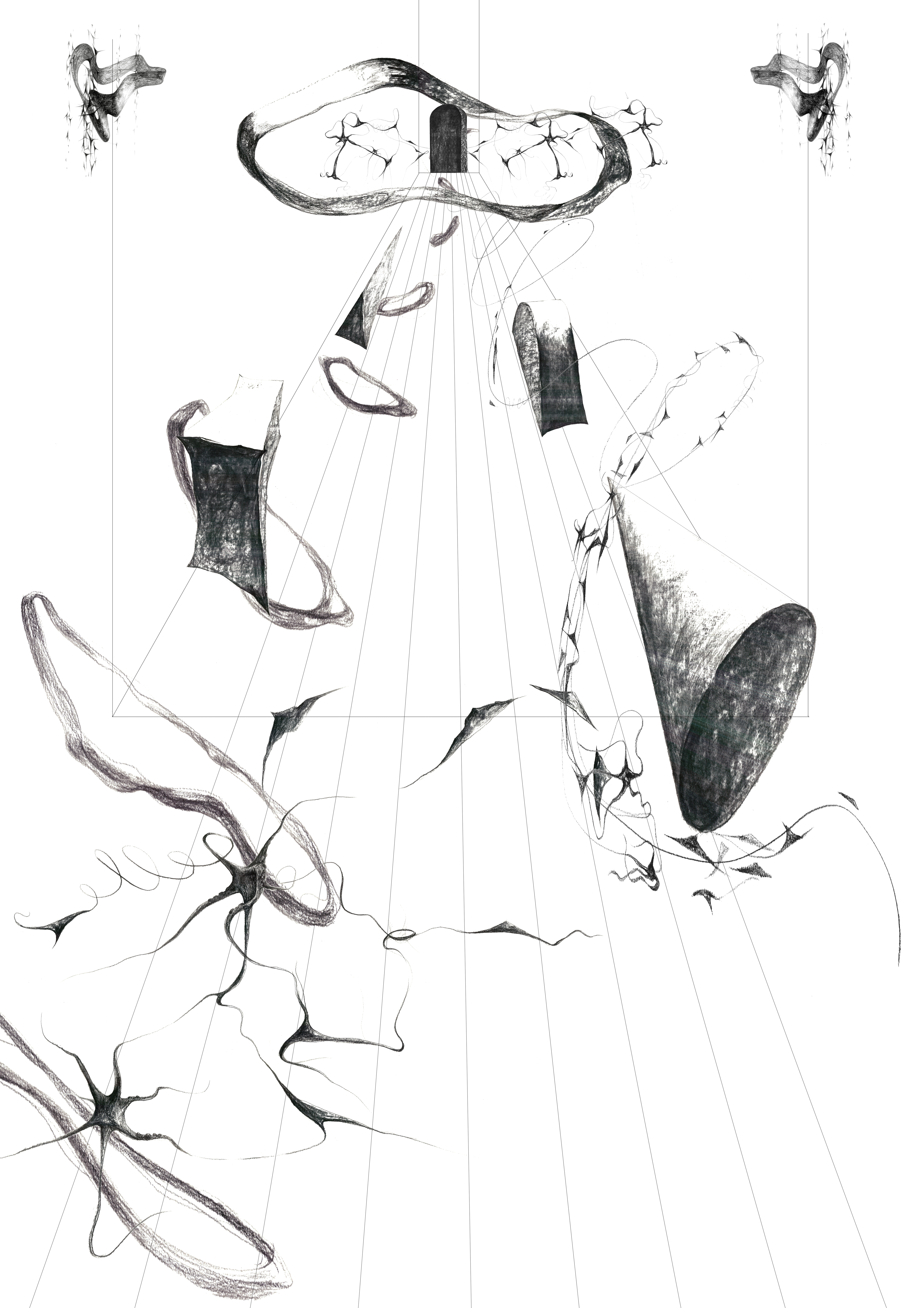
“Edge of Data”
(Paper)
2019.7 841mm*594mm Ink-jet printing
```java
/**
* 坐标
*/
public class Coordinate {
private static Random xRandom = new Random();
private static Random yRandom = new Random();
public int x;
public int y;
/**
* 创建随机坐标
*
* @return
*/
public synchronized static Coordinate createRandom() {
Coordinate coordinate = new Coordinate();
coordinate.x = xRandom.nextInt();
coordinate.y = yRandom.nextInt();
return coordinate;
}
public static long calcDistance(Coordinate coordinate1, Coordinate coordinate2) {
return (long) Math.sqrt(Math.pow(coordinate1.x - coordinate2.x, 2) + Math.pow(coordinate1.y - coordinate2.y, 2));
}
}
/**
* 情绪
*/
public interface Emotion {
/**
* 情绪表达
*/
void express();
/**
* 喜
*/
class Happy implements Emotion {
@Override
public void express() {
}
}
/**
* 怒
*/
class Angry implements Emotion {
@Override
public void express() {
}
}
/**
* 忧
*/
class Worry implements Emotion {
@Override
public void express() {
}
}
/**
* 惧
*/
class Fear implements Emotion {
@Override
public void express() {
}
}
/**
* 爱
*/
class Love implements Emotion {
@Override
public void express() {
}
}
/**
* 憎
*/
class Hate implements Emotion {
@Override
public void express() {
}
}
/**
* 欲
*/
class Desire implements Emotion {
@Override
public void express() {
}
}
/**
* 创建情绪
*/
class EmotionCreator {
private static Random random = new Random();
/**
* 随机创建情绪
* @return
*/
public synchronized static Emotion createRandomEmotion() {
return create(random.nextInt());
}
private static Emotion create(int emotion) {
emotion = emotion % 7;
if (emotion == 0) {
return new Happy();
} else if (emotion == 1) {
return new Angry();
} else if (emotion == 2) {
return new Worry();
} else if (emotion == 3) {
return new Fear();
} else if (emotion == 4) {
return new Love();
} else if (emotion == 5) {
return new Hate();
}
return new Desire();
}
}
}
/**
* 人
*/
public class Human {
/**
* 当前坐标
*/
private Coordinate unknow = Coordinate.createRandom();
/**
* 当前情绪
*/
private Emotion emotion = Emotion.EmotionCreator.createRandomEmotion();
/**
* 匹配随机数
*/
private Long matchedNumber;
/**
* 走出未知的下一步
*/
public void moveToNextStep() {
unknow = Coordinate.createRandom();
}
public Coordinate getCoordinate() {
return unknow;
}
public Long getMatchedNumber() {
return matchedNumber;
}
public void setMatchedNumber(Long matchedNumber) {
this.matchedNumber = matchedNumber;
}
}
/**
* 随机数池子
*/
public class RandomPool {
/**
* 存放随机数
*/
private static List availableNumbers = new CopyOnWriteArrayList<>();
private static Random indexRandom = new Random();
static {
// 初始化随机数
availableNumbers.add(1L);
availableNumbers.add(2L);
availableNumbers.add(3L);
availableNumbers.add(4L);
availableNumbers.add(5L);
}
/**
* 获取随机数,因为池子有限,所以可能获取不到
*/
@Nullable
public synchronized static Long getRandomNumber() {
if (availableNumbers.isEmpty()) {
return null;
}
int index = indexRandom.nextInt(availableNumbers.size());
return availableNumbers.remove(index);
}
}
/**
* 上帝管理着所有人
*/
public class God {
private static List allPeople = new CopyOnWriteArrayList<>();
/**
* 时间的开始
*/
private static long startTime;
/**
* 现在
*/
private static long nowTime;
/**
* 昨天
*/
private static long lastDayTime;
static {
// 记录时间的开始
startTime = System.currentTimeMillis();
Calendar calendar = Calendar.getInstance();
calendar.setTimeInMillis(startTime);
calendar.set(Calendar.MILLISECOND, 0);
calendar.set(Calendar.SECOND, 0);
calendar.set(Calendar.MINUTE, 0);
calendar.set(Calendar.HOUR_OF_DAY, 0);
lastDayTime = calendar.getTimeInMillis();
}
/**
* 造人
*
* @return
*/
public static Human turing() {
Human human = new Human();
allPeople.add(human);
return human;
}
/**
* 更新现在时间
*
* @param time
*/
public static void updateTime(long time) {
nowTime = time;
Calendar calendar = Calendar.getInstance();
calendar.setTimeInMillis(time);
calendar.set(Calendar.MILLISECOND, 0);
calendar.set(Calendar.SECOND, 0);
calendar.set(Calendar.MINUTE, 0);
calendar.set(Calendar.HOUR_OF_DAY, 0);
long todayTime = calendar.getTimeInMillis();
// 又一天
if (todayTime > lastDayTime) {
lastDayTime = todayTime;
// 大家往前走一步
for (Human person : allPeople) {
person.moveToNextStep();
}
// 开始算距离
for (int i = 0; i < allPeople.size(); i++) {
Human human1 = allPeople.get(i);
if (human1.getMatchedNumber() == null) {
// human1没有匹配到人
for (int i1 = 0; i1 < allPeople.size(); i1++) {
if (i != i1) {
Human human2 = allPeople.get(i1);
if (human2.getMatchedNumber() == null) {
// human2没有匹配到人
if (Coordinate.calcDistance(human1.getCoordinate(), human2.getCoordinate()) <= 45) {
// 两者距离小于等于45,设置匹配
Long randomNumber = RandomPool.getRandomNumber();
human1.setMatchedNumber(randomNumber);
human2.setMatchedNumber(randomNumber);
break;
}
}
}
}
}
}
}
}
}
/**
* 入口
*/
public class Entrance {
/**
* 时间
*/
private static Timer timer = new Timer();
private static TimerTask timerTask = new TimerTask() {
@Override
public void run() {
God.updateTime(System.currentTimeMillis());
}
};
public static void main(String[] args) {
// 时间开始运转
timer.schedule(timerTask, 0, 1000);
// 先造10个人
for (int i = 0; i < 10; i++) {
God.turing();
}
// 时间持续流逝
for (; ; ) {
}
}
}
```
(Coding by Rui Ou)
/**
* 坐标
*/
public class Coordinate {
private static Random xRandom = new Random();
private static Random yRandom = new Random();
public int x;
public int y;
/**
* 创建随机坐标
*
* @return
*/
public synchronized static Coordinate createRandom() {
Coordinate coordinate = new Coordinate();
coordinate.x = xRandom.nextInt();
coordinate.y = yRandom.nextInt();
return coordinate;
}
public static long calcDistance(Coordinate coordinate1, Coordinate coordinate2) {
return (long) Math.sqrt(Math.pow(coordinate1.x - coordinate2.x, 2) + Math.pow(coordinate1.y - coordinate2.y, 2));
}
}
/**
* 情绪
*/
public interface Emotion {
/**
* 情绪表达
*/
void express();
/**
* 喜
*/
class Happy implements Emotion {
@Override
public void express() {
}
}
/**
* 怒
*/
class Angry implements Emotion {
@Override
public void express() {
}
}
/**
* 忧
*/
class Worry implements Emotion {
@Override
public void express() {
}
}
/**
* 惧
*/
class Fear implements Emotion {
@Override
public void express() {
}
}
/**
* 爱
*/
class Love implements Emotion {
@Override
public void express() {
}
}
/**
* 憎
*/
class Hate implements Emotion {
@Override
public void express() {
}
}
/**
* 欲
*/
class Desire implements Emotion {
@Override
public void express() {
}
}
/**
* 创建情绪
*/
class EmotionCreator {
private static Random random = new Random();
/**
* 随机创建情绪
* @return
*/
public synchronized static Emotion createRandomEmotion() {
return create(random.nextInt());
}
private static Emotion create(int emotion) {
emotion = emotion % 7;
if (emotion == 0) {
return new Happy();
} else if (emotion == 1) {
return new Angry();
} else if (emotion == 2) {
return new Worry();
} else if (emotion == 3) {
return new Fear();
} else if (emotion == 4) {
return new Love();
} else if (emotion == 5) {
return new Hate();
}
return new Desire();
}
}
}
/**
* 人
*/
public class Human {
/**
* 当前坐标
*/
private Coordinate unknow = Coordinate.createRandom();
/**
* 当前情绪
*/
private Emotion emotion = Emotion.EmotionCreator.createRandomEmotion();
/**
* 匹配随机数
*/
private Long matchedNumber;
/**
* 走出未知的下一步
*/
public void moveToNextStep() {
unknow = Coordinate.createRandom();
}
public Coordinate getCoordinate() {
return unknow;
}
public Long getMatchedNumber() {
return matchedNumber;
}
public void setMatchedNumber(Long matchedNumber) {
this.matchedNumber = matchedNumber;
}
}
/**
* 随机数池子
*/
public class RandomPool {
/**
* 存放随机数
*/
private static List
private static Random indexRandom = new Random();
static {
// 初始化随机数
availableNumbers.add(1L);
availableNumbers.add(2L);
availableNumbers.add(3L);
availableNumbers.add(4L);
availableNumbers.add(5L);
}
/**
* 获取随机数,因为池子有限,所以可能获取不到
*/
@Nullable
public synchronized static Long getRandomNumber() {
if (availableNumbers.isEmpty()) {
return null;
}
int index = indexRandom.nextInt(availableNumbers.size());
return availableNumbers.remove(index);
}
}
/**
* 上帝管理着所有人
*/
public class God {
private static List
/**
* 时间的开始
*/
private static long startTime;
/**
* 现在
*/
private static long nowTime;
/**
* 昨天
*/
private static long lastDayTime;
static {
// 记录时间的开始
startTime = System.currentTimeMillis();
Calendar calendar = Calendar.getInstance();
calendar.setTimeInMillis(startTime);
calendar.set(Calendar.MILLISECOND, 0);
calendar.set(Calendar.SECOND, 0);
calendar.set(Calendar.MINUTE, 0);
calendar.set(Calendar.HOUR_OF_DAY, 0);
lastDayTime = calendar.getTimeInMillis();
}
/**
* 造人
*
* @return
*/
public static Human turing() {
Human human = new Human();
allPeople.add(human);
return human;
}
/**
* 更新现在时间
*
* @param time
*/
public static void updateTime(long time) {
nowTime = time;
Calendar calendar = Calendar.getInstance();
calendar.setTimeInMillis(time);
calendar.set(Calendar.MILLISECOND, 0);
calendar.set(Calendar.SECOND, 0);
calendar.set(Calendar.MINUTE, 0);
calendar.set(Calendar.HOUR_OF_DAY, 0);
long todayTime = calendar.getTimeInMillis();
// 又一天
if (todayTime > lastDayTime) {
lastDayTime = todayTime;
// 大家往前走一步
for (Human person : allPeople) {
person.moveToNextStep();
}
// 开始算距离
for (int i = 0; i < allPeople.size(); i++) {
Human human1 = allPeople.get(i);
if (human1.getMatchedNumber() == null) {
// human1没有匹配到人
for (int i1 = 0; i1 < allPeople.size(); i1++) {
if (i != i1) {
Human human2 = allPeople.get(i1);
if (human2.getMatchedNumber() == null) {
// human2没有匹配到人
if (Coordinate.calcDistance(human1.getCoordinate(), human2.getCoordinate()) <= 45) {
// 两者距离小于等于45,设置匹配
Long randomNumber = RandomPool.getRandomNumber();
human1.setMatchedNumber(randomNumber);
human2.setMatchedNumber(randomNumber);
break;
}
}
}
}
}
}
}
}
}
/**
* 入口
*/
public class Entrance {
/**
* 时间
*/
private static Timer timer = new Timer();
private static TimerTask timerTask = new TimerTask() {
@Override
public void run() {
God.updateTime(System.currentTimeMillis());
}
};
public static void main(String[] args) {
// 时间开始运转
timer.schedule(timerTask, 0, 1000);
// 先造10个人
for (int i = 0; i < 10; i++) {
God.turing();
}
// 时间持续流逝
for (; ; ) {
}
}
}
```
(Coding by Rui Ou)
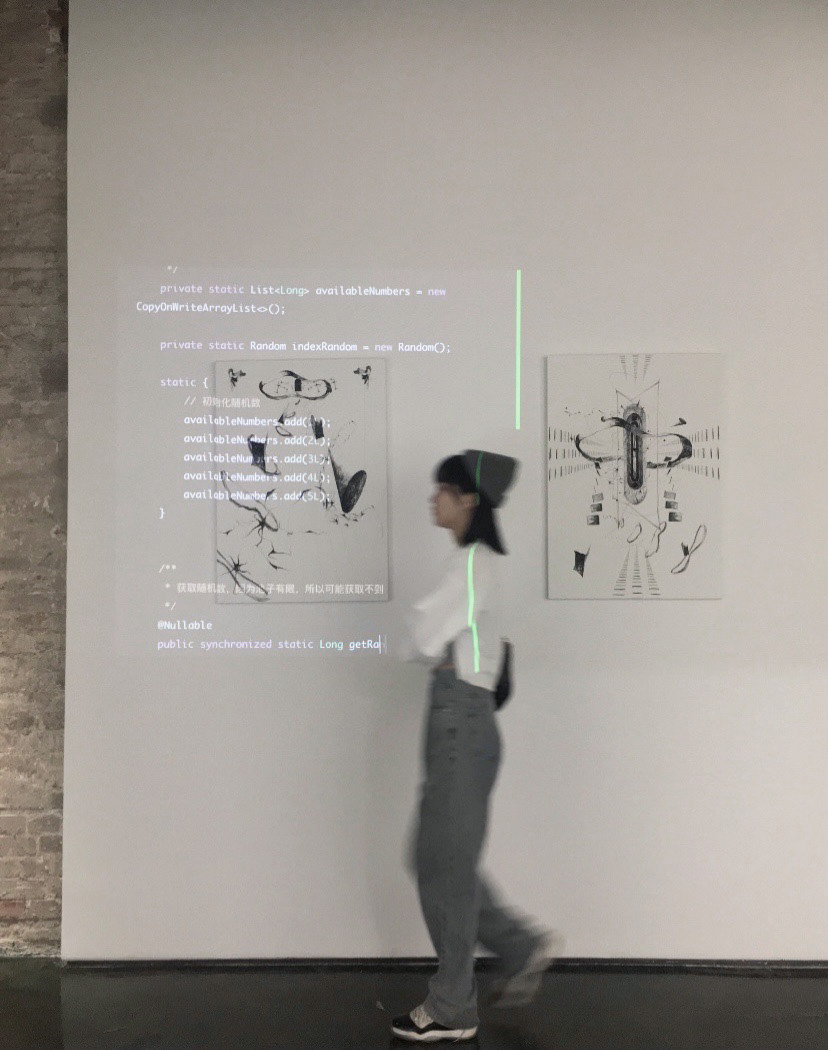
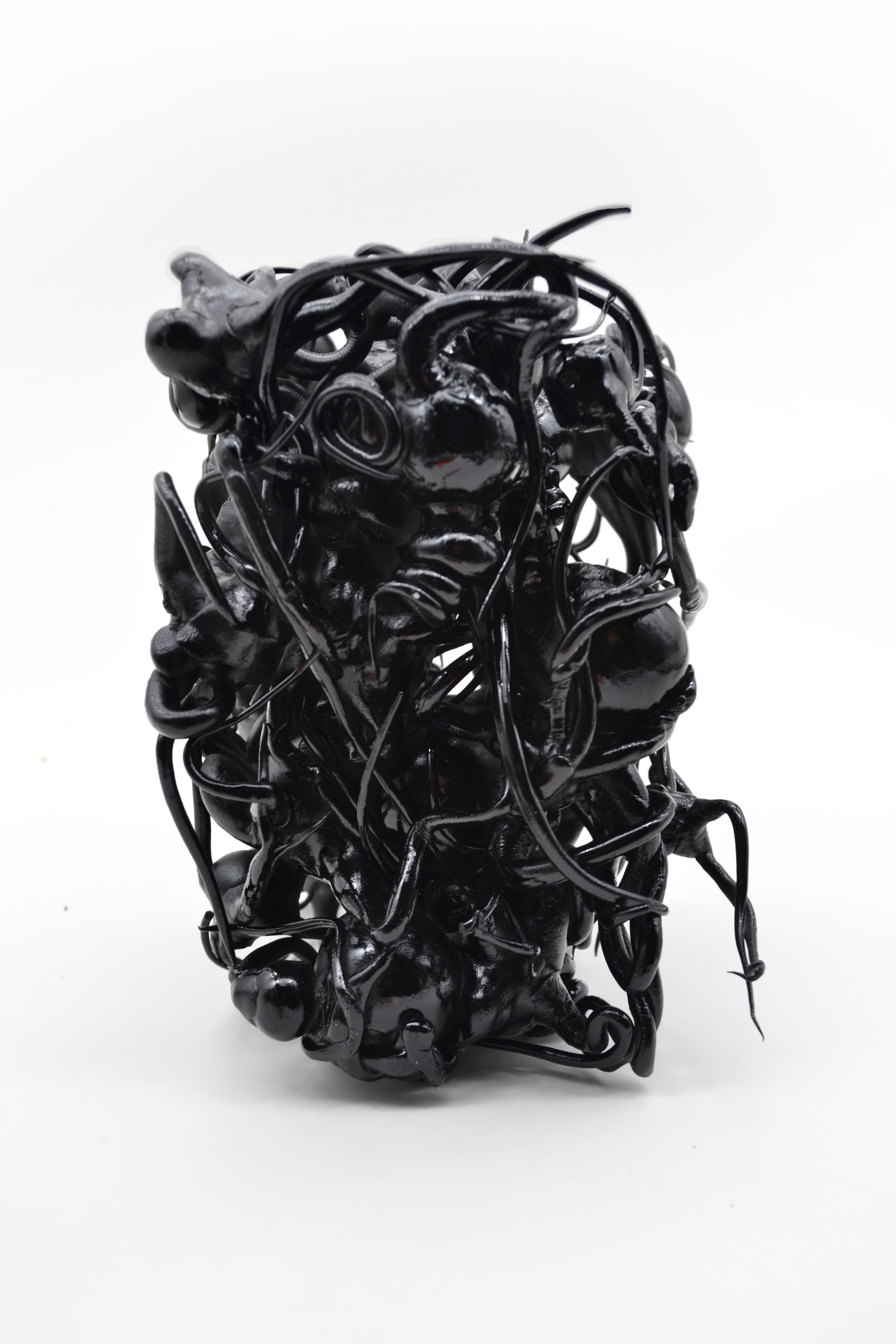
“Black Bastard”
(sculpture)
2019.10
256mm*203mm*177 clay